- Im trying to add two UIbuttons to a UITableViewCell, similar to the contacts app. Creating the UIButtons programmatically and adding them to the cell’s subview is trivial. However the interface is a bit different from the contacts app, see image here…
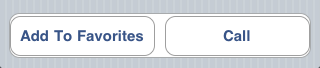
cell = [[[UITableViewCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:@"ButtonCell"] autorelease];
UIButton *buttonLeft = [UIButton buttonWithType:UIButtonTypeRoundedRect];
[buttonLeft setTitle:@"Left" forState:UIControlStateNormal];
[buttonLeft setFrame: CGRectMake( 10.0f, 3.0f, 145.0f, 40.0f)];
[buttonLeft addTarget:self action:@selector(addToFavorites) forControlEvents:UIControlEventTouchUpInside];
UIButton *buttonRight = [UIButton buttonWithType:UIButtonTypeRoundedRect];
[buttonRight setTitle:@"Right" forState:UIControlStateNormal];
[buttonRight setFrame: CGRectMake( 165.0f, 3.0f, 145.0f, 40.0f)];
[buttonRight addTarget:self action:@selector(addToFavorites) forControlEvents:UIControlEventTouchUpInside];
[cell addSubview:buttonLeft];
[cell addSubview:buttonRight];
|
- So to make the background the same as UITableViewStyleGrouped, we can implement:
cell.backgroundColor = [UIColor groupTableViewBackgroundColor];
|
But I could still see the border of the UITableViewCell.. see image below…

- The crux of it was to implement a UIView, set the background as UITableViewStyleGrouped and add that as a cell’s backgroundView.
cell = [[[UITableViewCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:@"ButtonCell"] autorelease];
UIView *backgroundView = [[UIView alloc] initWithFrame:CGRectMake(0.0f, 0.0f, 320.0f, 44.0f)];
backgroundView.backgroundColor = [UIColor groupTableViewBackgroundColor];
cell.backgroundView = backgroundView;
UIButton *buttonLeft = [UIButton buttonWithType:UIButtonTypeRoundedRect];
[buttonLeft setTitle:@"Left" forState:UIControlStateNormal];
[buttonLeft setFrame: CGRectMake( 10.0f, 3.0f, 145.0f, 40.0f)];
[buttonLeft addTarget:self action:@selector(addToFavorites) forControlEvents:UIControlEventTouchUpInside];
UIButton *buttonRight = [UIButton buttonWithType:UIButtonTypeRoundedRect];
[buttonRight setTitle:@"Right" forState:UIControlStateNormal];
[buttonRight setFrame: CGRectMake( 165.0f, 3.0f, 145.0f, 40.0f)];
[buttonRight addTarget:self action:@selector(addToFavorites) forControlEvents:UIControlEventTouchUpInside];
[cell addSubview:buttonLeft];
[cell addSubview:buttonRight];
[backgroundView release];
|
Now the interface is a whole lot cleaner and the resulting image is better…
